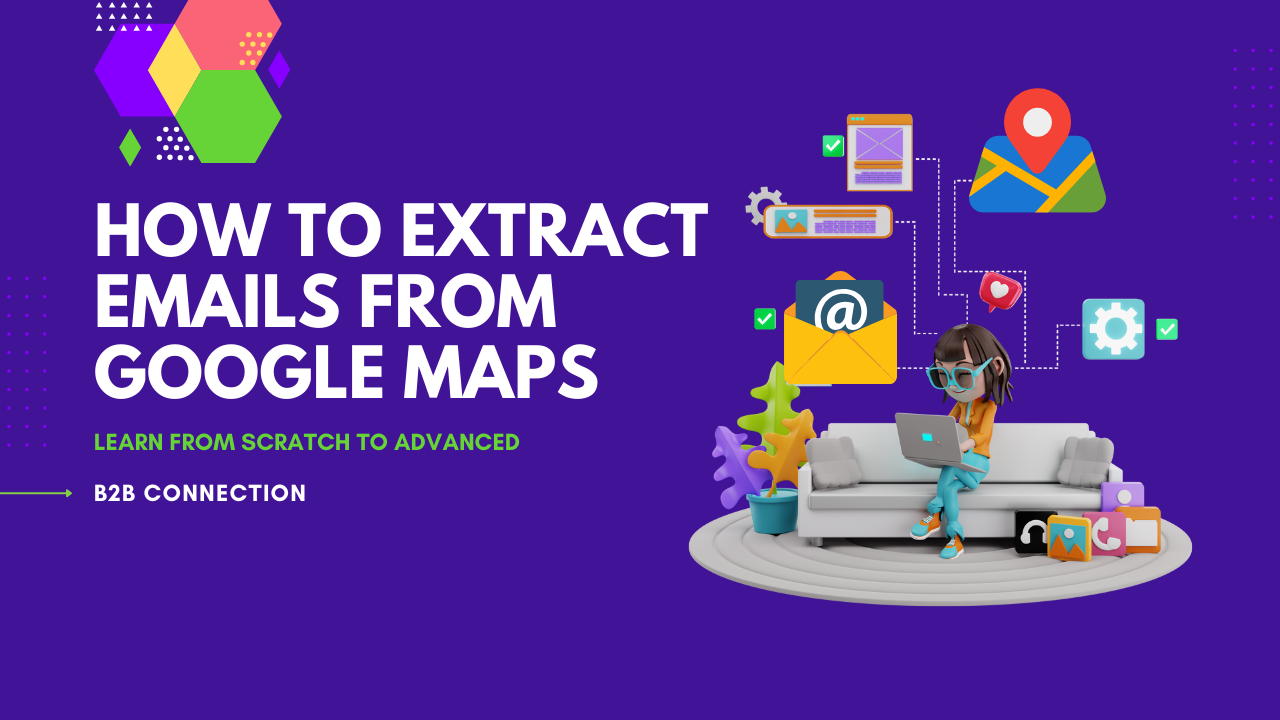
Table of Contents
In today’s digital age, extracting email addresses from Google Maps can be a valuable strategy for businesses looking to expand their outreach and connect with potential customers. This blog post will guide you through a two-step process to scrape emails from Google Maps, which involves first scraping Google Maps data and then extracting emails from the websites associated with the scraped data. Let’s dive in!
Step 1: Scraping Google Maps Data
The first step in this process is to collect data from Google Maps. This involves extracting information such as business names, addresses, phone numbers, and websites. Here’s how you can do it:
1. Setting Up the Environment:
- Install Python and necessary libraries:
pip install requests beautifulsoup4 selenium
Configure a web driver for Selenium (e.g., ChromeDriver) and ensure it matches your browser version.
2. Writing the Scraping Script:
- Use Selenium to navigate Google Maps and extract data. Below is a basic example of how to set up Selenium for this task:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
# Initialize the WebDriver
driver = webdriver.Chrome(executable_path='path/to/chromedriver')
# Open Google Maps
driver.get('https://www.google.com/maps')
# Search for a specific type of business in a location
search_box = driver.find_element(By.ID, 'searchboxinput')
search_box.send_keys('restaurants in New York')
search_box.send_keys(Keys.RETURN)
time.sleep(5) # Allow time for search results to load
# Scrape business details
businesses = driver.find_elements(By.CLASS_NAME, 'section-result')
business_data = []
for business in businesses:
name = business.find_element(By.CLASS_NAME, 'section-result-title').text
try:
website = business.find_element(By.CLASS_NAME, 'section-result-action-item').get_attribute('href')
except:
website = None
business_data.append({'name': name, 'website': website})
driver.quit()
# Save data to a file or database
with open('google_maps_data.json', 'w') as file:
json.dump(business_data, file)
3. Handling Anti-Scraping Mechanisms:
- Google Maps may have anti-scraping mechanisms. To mitigate this, consider implementing rotating proxies, varying your scraping patterns, and adhering to ethical scraping practices.
Step 2: Scraping Emails from the Scraped Google Maps Data Websites
Once you have the business data from Google Maps, the next step is to visit each business’s website and extract email addresses. Hereβs how you can achieve this:
1. Setting Up for Email Extraction:
- Install additional libraries if needed:
pip install requests beautifulsoup4
2. Writing the Email Extraction Script:
- Use BeautifulSoup to parse the HTML of the websites and extract email addresses. Hereβs a basic script to get you started:
import requests
from bs4 import BeautifulSoup
import re
# Load the business data
with open('google_maps_data.json', 'r') as file:
business_data = json.load(file)
email_pattern = re.compile(r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b')
for business in business_data:
if business['website']:
try:
response = requests.get(business['website'])
soup = BeautifulSoup(response.text, 'html.parser')
emails = set(re.findall(email_pattern, soup.text))
business['emails'] = list(emails)
except:
business['emails'] = []
# Save updated data with emails
with open('google_maps_data_with_emails.json', 'w') as file:
json.dump(business_data, file)
3. Ethical Considerations and Legal Compliance:
- Ensure that your scraping activities comply with legal regulations and the terms of service of the websites you are scraping. Always prioritize ethical scraping practices.
Conclusion
Scraping emails from Google Maps can be a powerful tool for business outreach. By following the two-step process outlined in this blog, you can efficiently gather email addresses from businesses listed on Google Maps. Remember to adhere to ethical and legal standards to ensure that your scraping activities are responsible and compliant.
If you have any questions or need further assistance with web scraping, feel free to reach out to us at B2B Connection. Happy scraping!